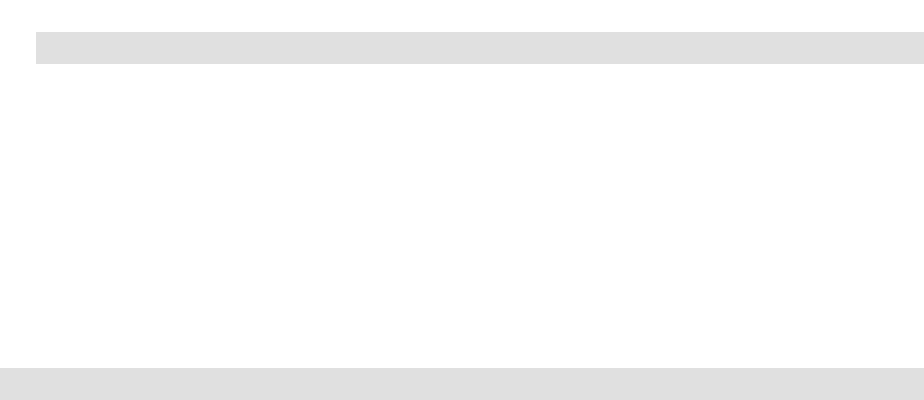
printf("Element found at position: %d", (i +
1));
break;
}
}
}
int main() {
int arr[] = { 1, 2, 3, 4, 5, 6 };
// no of elements
int n = 6;
// key to be searched
int key = 3;
// Calling function to find the key
findElement(arr, n, key);
return 0;
}
Output:
Element found at position: 3
3. C continue: This loop control statement is just like the break
statement. The continue statement is opposite to that of
break statement, instead of terminating the loop, it forces to execute
the next iteration of the loop.
As the name suggest the continue statement forces the loop to
continue or execute the next iteration. When the continue statement is
executed in the loop, the code inside the loop following the continue
statement will be skipped and next iteration of the loop will begin.
Syntax:
4. continue;